Note: There is an improved version in the next post.
Following my earlier post about implementing a REST API, here is a simple listener for NetBox webhooks:
webhook-listener.py:
import logging from flask import Flask, request from flask_restplus import Api, Resource, fields app = Flask(__name__) api = Api(app, version="1.0", title="NetBox Webhook Listener", description="Tested with NetBox 2.6.6") ns = api.namespace("webhook") APP_NAME = "webhook-listener" logger = logging.getLogger(APP_NAME) logger.setLevel(logging.INFO) formatter = logging.Formatter("%(asctime)s %(name)s %(levelname)s: %(message)s") file_logging = logging.FileHandler("{}.log".format(APP_NAME)) file_logging.setFormatter(formatter) logger.addHandler(file_logging) webhook_request = api.model("Webhook request from NetBox", { 'username': fields.String, 'data': fields.Raw(description="The object data from NetBox"), 'event': fields.String, 'timestamp': fields.String, 'model': fields.String, 'request_id': fields.String, }) def do_something_with_the_event(data): pass @ns.route("/") class WebhookListener(Resource): @ns.expect(webhook_request) def post(self): try: input_data = request.json except: input_data = {} logger.info("{}".format(input_data)) if not input_data or "model" not in input_data: return {"result":"invalid input"}, 400 do_something_with_the_event(input_data) return {"result":"ok"}, 200 if __name__ == "__main__": app.run(host="0.0.0.0")
With the other configurations described in the earlier post (and after configured NetBox to send the webhook POST requests to http://my.server.local/webhook/
), these are the outputs:
webhook-listener.log:
2019-10-12 18:16:12,781 webhook-listener INFO: {'username': 'admin', 'data': {'id': 7, 'description': '', 'slug': 'my-testing-tenant', 'name': 'My Testing Tenant', 'group': None, 'comments': '', 'tags': ['Finland', 'Torilla tavataan'], 'last_updated': '2019-10-12T15:16:12.641722Z', 'created': '2019-10-12', 'custom_fields': {}}, 'event': 'created', 'timestamp': '2019-10-12 15:16:12.761427', 'model': 'tenant', 'request_id': '733486ff-987b-4cb1-bb2d-dd5c3c7d6b04'}
Automatically generated Swagger API documentation on http://my.server.local/
:
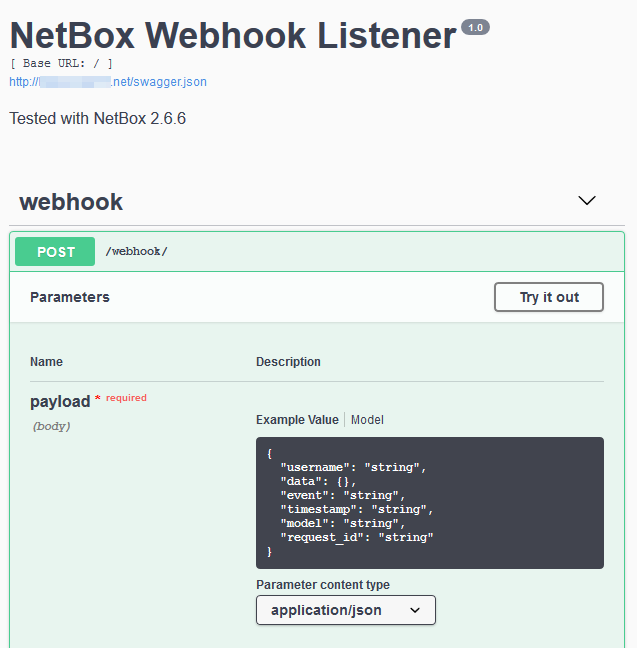
Excellent example!